Animation Creation Ideas
1. Using time.sleep() of the time module
2. Using os module
3. FuncAnimation
The first two ideas are very well known methods. I also tried to implement animation through two ideas, but it was not suitable for colab environment and it was difficult to write code friendly to pyplot module. I was able to check the technical documentation about FuncAnimation of the matplotlib module and solved the animation creation problem using that method.
If you have other ideas, please leave a comment.
matplotlib.animation
Inheritance Diagrams
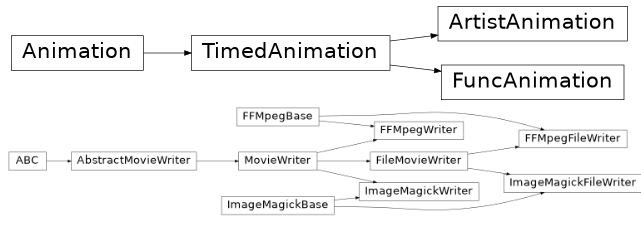
Animation#
The easiest way to make a live animation in Matplotlib is to use one of the Animation
classes.
Animation |
A base class for Animations. |
FuncAnimation |
Makes an animation by repeatedly calling a function func. |
ArtistAnimation |
Animation using a fixed set of Artist objects. |
In both cases it is critical to keep a reference to the instance object. The animation is advanced by a timer (typically from the host GUI framework) which the Animation
object holds the only reference to. If you do not hold a reference to the Animation
object, it (and hence the timers) will be garbage collected which will stop the animation.
To save an animation use Animation.save
, Animation.to_html5_video
, or Animation.to_jshtml
.
See Helper Classes below for details about what movie formats are supported.
FuncAnimation
#
The inner workings of FuncAnimation
is more-or-less:
for d in frames:
artists = func(d, *fargs)
fig.canvas.draw_idle()
fig.canvas.start_event_loop(interval)
with details to handle 'blitting' (to dramatically improve the live performance), to be non-blocking, not repeatedly start/stop the GUI event loop, handle repeats, multiple animated axes, and easily save the animation to a movie file.
'Blitting' is a standard technique in computer graphics. The general gist is to take an existing bit map (in our case a mostly rasterized figure) and then 'blit' one more artist on top. Thus, by managing a saved 'clean' bitmap, we can only re-draw the few artists that are changing at each frame and possibly save significant amounts of time. When we use blitting (by passing blit=True
), the core loop of FuncAnimation
gets a bit more complicated:
ax = fig.gca()
def update_blit(artists):
fig.canvas.restore_region(bg_cache)
for a in artists:
a.axes.draw_artist(a)
ax.figure.canvas.blit(ax.bbox)
artists = init_func()
for a in artists:
a.set_animated(True)
fig.canvas.draw()
bg_cache = fig.canvas.copy_from_bbox(ax.bbox)
for f in frames:
artists = func(f, *fargs)
update_blit(artists)
fig.canvas.start_event_loop(interval)
This is of course leaving out many details (such as updating the background when the figure is resized or fully re-drawn). However, this hopefully minimalist example gives a sense of how init_func
and func
are used inside of FuncAnimation
and the theory of how 'blitting' works.
The expected signature on func
and init_func
is very simple to keep FuncAnimation
out of your book keeping and plotting logic, but this means that the callable objects you pass in must know what artists they should be working on. There are several approaches to handling this, of varying complexity and encapsulation. The simplest approach, which works quite well in the case of a script, is to define the artist at a global scope and let Python sort things out. For example
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
xdata, ydata = [], []
ln, = plt.plot([], [], 'ro')
def init():
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
return ln,
def update(frame):
xdata.append(frame)
ydata.append(np.sin(frame))
ln.set_data(xdata, ydata)
return ln,
ani = FuncAnimation(fig, update, frames=np.linspace(0, 2*np.pi, 128),
init_func=init, blit=True)
plt.show()
The second method is to use functools.partial
to 'bind' artists to function. A third method is to use closures to build up the required artists and functions. A fourth method is to create a class.
'학부공부 > OS_운영체제' 카테고리의 다른 글
PE1. Practice Exercises_2.1~2.8_Text book 101p (2) | 2022.07.10 |
---|---|
TP1.9. Python Part Executable File Excessive Execution Time Problem [OS Scheduling simulator] (2) | 2022.06.06 |
TP1.7. History in Colab shared links [OS Scheduling simulator] (2) | 2022.06.06 |
TP1.6. Corner case [OS Scheduling simulator] (2) | 2022.06.06 |
TP1.5. File format for Evaluation module [OS Scheduling simulator] (2) | 2022.06.06 |
댓글